use the Compare() method to compare DateTime instances
using System;
class MainClass {
public static void Main() {
DateTime myDateTime = DateTime.Now;
DateTime myDateTime2 = DateTime.UtcNow;
DateTime myDateTime3 = new DateTime(2004, 1, 13);
DateTime myDateTime4 = new DateTime(2004, 1, 14);
Console.WriteLine("myDateTime3 = " + myDateTime3);
Console.WriteLine("myDateTime4 = " + myDateTime4);
int intResult = DateTime.Compare(myDateTime3, myDateTime4);
Console.WriteLine("DateTime.Compare(myDateTime3,myDateTime4) = " + DateTime.Compare(myDateTime, myDateTime2));
}
}
Related examples in the same category
1. | Current date and time | | |
2. | What day of the month is this? | | |
3. | Do some leap year checks | | |
4. | Look at the min and max date/time values | | |
5. | Output DateTime object | | |
6. | Constructors of DateTime | | |
7. | comparisons between DateTime objects | | |
8. | Parse and ParseExact | | |
9. | DateTime Now and its calculation | | |
10. | new DateTime(1900, 2, 29) | | |
11. | new DateTime(1900, 2, 29, new JulianCalendar()) | | |
12. | Specify Kind DateTime | | |
13. | Offset of DateTime | | |
14. | DateTime and TimeSpan Instances | | |
15. | use the Now and UtcNow properties to get the currrent date and time | | |
16. | display the Date, Day, DayOfWeek, DayOfYear,Ticks, and TimeOfDayProperties of myDateTime | | |
17. | use the overloaded less than operator (<) to compare two DateTime instances | | |
18. | use the Equals() method to compare DateTime instances | | |
19. | use the DaysInMonth() method to retrieve the number of days in a particular month and year | | |
20. | use the IsLeapYear() method to determine if a particular year is a leap year | | |
21. | use the Parse() method to convert strings to DateTime instances | | |
22. | use the Subtract() method to subtract a TimeSpan from a DateTime | | |
23. | use the overloaded subtraction operator (-) to subtract a TimeSpan from a DateTime | | |
24. | Illustrates the use of DateTime and TimeSpan instances | | 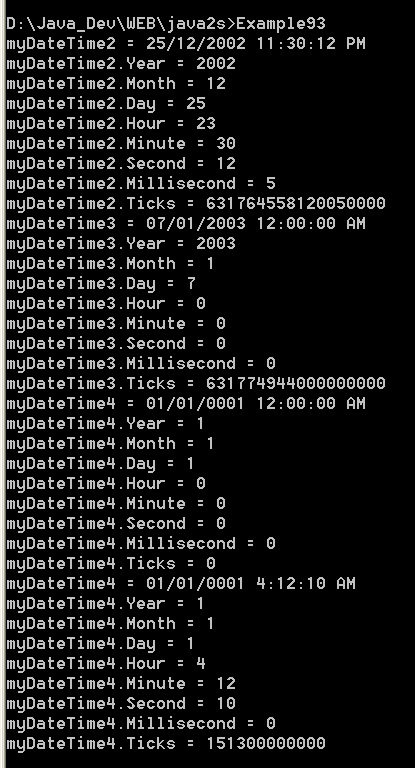 |
25. | Displays the words 'Hello World!' on the screen, along with the current date and time | |  |
26. | illustrates the use of TimeSpan properties and methods | | 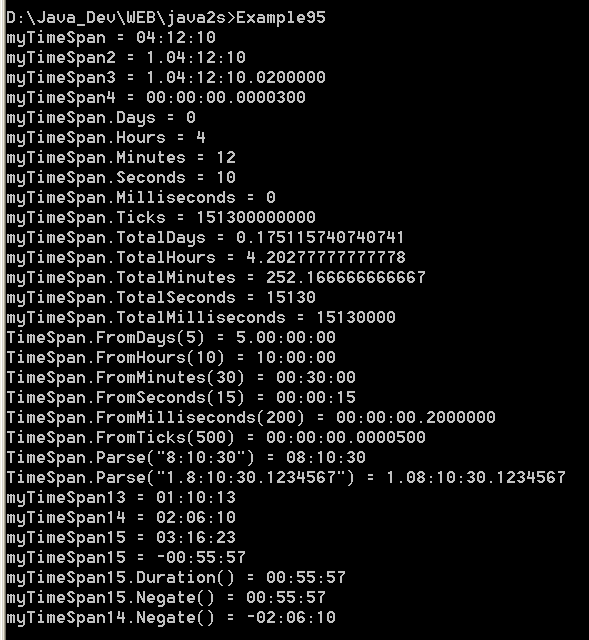 |
27. | A simple clock | | 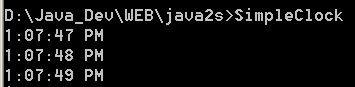 |
28. | Estimates pi by throwing points into a square. Use to
compare execution times | |  |
29. | Change current culture and back | | |
30. | How values you passed into contructor mapped to its Properties | | |
31. | Gets the day of the week | | |
32. | Returns the number of days in the specified month and year. | | |
33. | DateTime and == | | |
34. | Create a DateTime structure with local time | | |
35. | Compare DateTime instance with Equal methods | | |
36. | Format a DateTime with M/dd/yyyy h:mm:ss.fff tt | | |
37. | Check the Kind of DateTime | | |
38. | DateTimeFormatInfo.CurrentInfo.TimeSeparator | | |
39. | Initializes a DateTime structure to a specified number of ticks. | | |
40. | DateTime.Now, DateTime.UtcNow, DateTime.Today | | |
41. | Parse a date time value from a string with Invariant Culture | | |
42. | Default value of DateTime is DateTime.MinValue | | |
43. | Default ToString output | | |
44. | Convert DateTime to string with fr-FR culture | | |
45. | ToString single letter format: F | | |
46. | DateTime ToString single letter format with fr-FR culture | | |
47. | DateTime Compare | | |
48. | DateTime CompareTo | | |
49. | Gets the date component of this instance. | | |
50. | Time String | | |
51. | Create day and year for a certain month | | |
52. | Returns the number of seconds since January 1, 1970 | | |