Measures the time taken to add some numbers
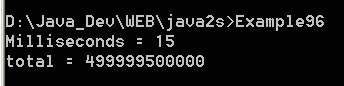
/*
Mastering Visual C# .NET
by Jason Price, Mike Gunderloy
Publisher: Sybex;
ISBN: 0782129110
*/
/*
Example9_6.cs measures the time taken to add some numbers
*/
using System;
public class Example9_6
{
public static void Main()
{
// create a DateTime object and set it to the
// current date and time
DateTime start = DateTime.Now;
// add numbers using a for loop
long total = 0;
for (int count = 0; count < 1000000; count++)
{
total += count;
}
// subtract the current date and time from the start,
// storing the difference in a TimeSpan
TimeSpan timeTaken = DateTime.Now - start;
// display the number of milliseconds taken to add the numbers
Console.WriteLine("Milliseconds = " + timeTaken.Milliseconds);
// display the total of the added numbers
Console.WriteLine("total = " + total);
}
}
Related examples in the same category