Get specified column data type and column name from OleDbSchemaTable
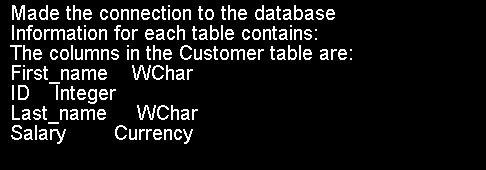
using System;
using System.Data;
using System.Data.OleDb;
public class DatabaseInfo {
public static void Main () {
String connect = "Provider=Microsoft.JET.OLEDB.4.0;data source=.\\Employee.mdb";
OleDbConnection con = new OleDbConnection(connect);
con.Open();
Console.WriteLine("Made the connection to the database");
Console.WriteLine("Information for each table contains:");
DataTable tables = con.GetOleDbSchemaTable(OleDbSchemaGuid.Tables,new object[]{null,null,null,"TABLE"});
DataTable cols = con.GetOleDbSchemaTable(OleDbSchemaGuid.Columns,
new object[]{null,null,"Employee",null});
Console.WriteLine("The columns in the Customer table are:");
foreach(DataRow row in cols.Rows)
Console.WriteLine(" {0}\t{1}", row[3],(OleDbType)row[11]);
con.Close();
}
}
Related examples in the same category