ADO.NET Binding: Master Detail
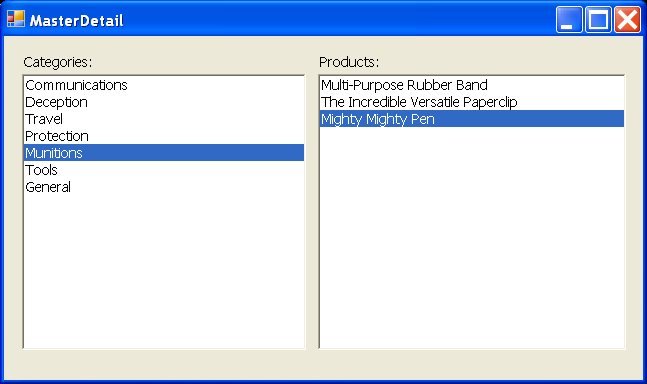
/*
User Interfaces in C#: Windows Forms and Custom Controls
by Matthew MacDonald
Publisher: Apress
ISBN: 1590590457
*/
using System;
using System.Data;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
namespace ADO.NET_Binding
{
/// <summary>
/// Summary description for MasterDetail.
/// </summary>
public class MasterDetail : System.Windows.Forms.Form
{
internal System.Windows.Forms.Label Label2;
internal System.Windows.Forms.Label Label1;
internal System.Windows.Forms.ListBox lstProduct;
internal System.Windows.Forms.ListBox lstCategory;
/// <summary>
/// Required designer variable.
/// </summary>
private System.ComponentModel.Container components = null;
public MasterDetail()
{
//
// Required for Windows Form Designer support
//
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent call
//
}
/// <summary>
/// Clean up any resources being used.
/// </summary>
protected override void Dispose( bool disposing )
{
if( disposing )
{
if(components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
#region Windows Form Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
this.Label2 = new System.Windows.Forms.Label();
this.Label1 = new System.Windows.Forms.Label();
this.lstProduct = new System.Windows.Forms.ListBox();
this.lstCategory = new System.Windows.Forms.ListBox();
this.SuspendLayout();
//
// Label2
//
this.Label2.Location = new System.Drawing.Point(212, 13);
this.Label2.Name = "Label2";
this.Label2.Size = new System.Drawing.Size(120, 16);
this.Label2.TabIndex = 9;
this.Label2.Text = "Products:";
//
// Label1
//
this.Label1.Location = new System.Drawing.Point(12, 13);
this.Label1.Name = "Label1";
this.Label1.Size = new System.Drawing.Size(120, 16);
this.Label1.TabIndex = 8;
this.Label1.Text = "Categories:";
//
// lstProduct
//
this.lstProduct.Location = new System.Drawing.Point(212, 29);
this.lstProduct.Name = "lstProduct";
this.lstProduct.Size = new System.Drawing.Size(208, 225);
this.lstProduct.TabIndex = 7;
//
// lstCategory
//
this.lstCategory.Location = new System.Drawing.Point(12, 29);
this.lstCategory.Name = "lstCategory";
this.lstCategory.Size = new System.Drawing.Size(192, 225);
this.lstCategory.TabIndex = 6;
//
// MasterDetail
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 14);
this.ClientSize = new System.Drawing.Size(432, 266);
this.Controls.AddRange(new System.Windows.Forms.Control[] {
this.Label2,
this.Label1,
this.lstProduct,
this.lstCategory});
this.Font = new System.Drawing.Font("Tahoma", 8.25F, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, ((System.Byte)(0)));
this.Name = "MasterDetail";
this.Text = "MasterDetail";
this.Load += new System.EventHandler(this.MasterDetail_Load);
this.ResumeLayout(false);
}
#endregion
private BindingManagerBase categoryBinding;
private DataSet dsStore = new DataSet();
private void MasterDetail_Load(object sender, System.EventArgs e)
{
dsStore.ReadXmlSchema(Application.StartupPath + "\\store.xsd");
dsStore.ReadXml(Application.StartupPath + "\\store.xml");
lstCategory.DataSource = dsStore.Tables["Categories"];
lstCategory.DisplayMember = "CategoryName";
lstProduct.DataSource = dsStore.Tables["Products"];
lstProduct.DisplayMember = "ModelName";
categoryBinding = this.BindingContext[dsStore.Tables["Categories"]];
categoryBinding.PositionChanged += new EventHandler(Binding_PositionChanged);
// Invoke method once to update child table at startup.
Binding_PositionChanged(null,null);
}
private void Binding_PositionChanged(object sender, System.EventArgs e)
{
string filter;
DataRow selectedRow;
// Find the current category row.
selectedRow = dsStore.Tables["Categories"].Rows[categoryBinding.Position];
// Create a filter expression using its CategoryID.
filter = "CategoryID='" + selectedRow["CategoryID"].ToString() + "'";
// Modify the view onto the product table.
dsStore.Tables["Products"].DefaultView.RowFilter = filter;
}
[STAThread]
static void Main()
{
Application.Run(new MasterDetail());
}
}
}
ADO.NETBinding.zip( 76 k)Related examples in the same category