illustrates casting objects
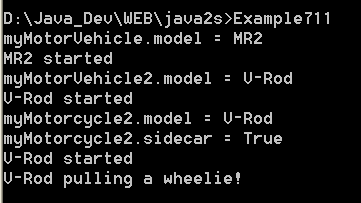
/*
Mastering Visual C# .NET
by Jason Price, Mike Gunderloy
Publisher: Sybex;
ISBN: 0782129110
*/
/*
Example7_11.cs illustrates casting objects
*/
using System;
// declare the MotorVehicle class (the base class)
class MotorVehicle
{
public string model;
public MotorVehicle(string model)
{
this.model = model;
}
public void Start()
{
Console.WriteLine(model + " started");
}
}
// declare the Car class
class Car : MotorVehicle
{
public bool convertible;
public Car(string model, bool convertible) :
base(model)
{
this.convertible = convertible;
}
}
// declare the Motorcycle class
class Motorcycle : MotorVehicle
{
public bool sidecar;
// define a constructor
public Motorcycle(string model, bool sidecar) :
base(model)
{
this.sidecar = sidecar;
}
public void PullWheelie()
{
Console.WriteLine(model + " pulling a wheelie!");
}
}
public class Example7_11
{
public static void Main()
{
// create a Car object
Car myCar = new Car("MR2", true);
// cast myCar to MotorVehicle (upcast)
MotorVehicle myMotorVehicle = (MotorVehicle) myCar;
// myMotorVehicle only has a model field and Start() method
// (no convertible field)
Console.WriteLine("myMotorVehicle.model = " + myMotorVehicle.model);
myMotorVehicle.Start();
// Console.WriteLine("myMotorVehicle.convertible = " +
// myMotorVehicle.convertible);
// create a Motorcycle object
Motorcycle myMotorcycle = new Motorcycle("V-Rod", true);
// cast myMotorcycle to MotorVehicle (upcast)
MotorVehicle myMotorVehicle2 = (MotorVehicle) myMotorcycle;
// myMotorVehicle only has a model field and Start() method
// (no sidecar field or PullWheelie() method)
Console.WriteLine("myMotorVehicle2.model = " + myMotorVehicle2.model);
myMotorVehicle2.Start();
// Console.WriteLine("myMotorVehicle2.sidecar = " +
// myMotorVehicle2.sidecar);
// myMotorVehicle2.PullWheelie();
// cast myMotorVehicle2 to Motorcycle (downcast)
Motorcycle myMotorcycle2 = (Motorcycle) myMotorVehicle2;
// myMotorCycle2 has access to all members of the Motorcycle class
Console.WriteLine("myMotorcycle2.model = " + myMotorcycle2.model);
Console.WriteLine("myMotorcycle2.sidecar = " + myMotorcycle2.sidecar);
myMotorcycle2.Start();
myMotorcycle2.PullWheelie();
// cannot cast a Motorcyle object to the Car class because
// their classes are not compatible
// Car myCar2 = (Car) myMotorVehicle2;
}
}
Related examples in the same category