illustrates the use of ArrayLists 2
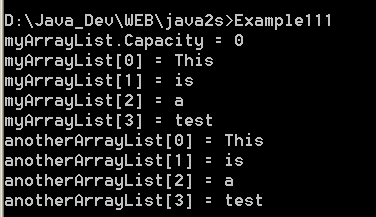
/*
Mastering Visual C# .NET
by Jason Price, Mike Gunderloy
Publisher: Sybex;
ISBN: 0782129110
*/
/*
Example11_1.cs illustrates the use of ArrayLists
*/
using System;
using System.Collections;
public class Example11_1
{
// the DisplayArrayList() method displays the elements in the
// ArrayList that is supplied as a parameter
public static void DisplayArrayList(
string arrayListName, ArrayList myArrayList
)
{
for (int counter = 0; counter < myArrayList.Count; counter++)
{
Console.WriteLine(arrayListName + "[" + counter + "] = " +
myArrayList[counter]);
}
}
public static void Main()
{
// create an ArrayList object
ArrayList myArrayList = new ArrayList();
// display the Capacity property
Console.WriteLine("myArrayList.Capacity = " +
myArrayList.Capacity);
// add four strings to myArrayList using the Add() method
myArrayList.Add("This");
myArrayList.Add("is");
myArrayList.Add("a");
myArrayList.Add("test");
// display the contents of myArrayList using DisplayArrayList()
DisplayArrayList("myArrayList", myArrayList);
// create another ArrayList, passing myArrayList to the
// constructor of the new ArrayList
ArrayList anotherArrayList = new ArrayList(myArrayList);
// display the contents of anotherArrayList
DisplayArrayList("anotherArrayList", anotherArrayList);
}
}
Related examples in the same category