Array reverse and sort
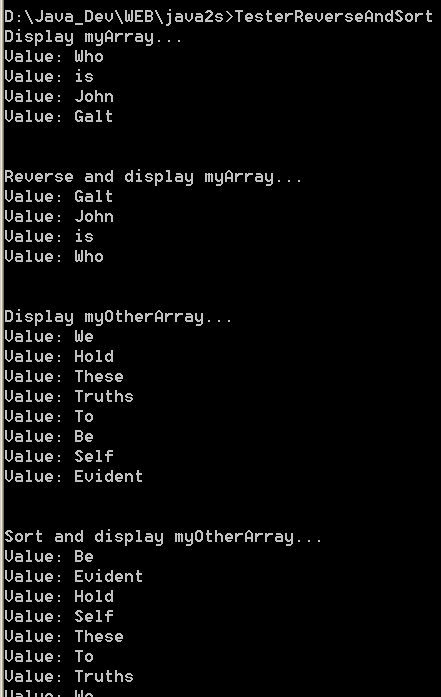
/*
Learning C#
by Jesse Liberty
Publisher: O'Reilly
ISBN: 0596003765
*/
using System;
namespace ReverseAndSort
{
public class TesterReverseAndSort
{
public static void DisplayArray(object[] theArray)
{
foreach (object obj in theArray)
{
Console.WriteLine("Value: {0}", obj);
}
Console.WriteLine("\n");
}
public void Run()
{
String[] myArray =
{
"Who", "is", "John", "Galt"
};
Console.WriteLine("Display myArray...");
DisplayArray(myArray);
Console.WriteLine("Reverse and display myArray...");
Array.Reverse(myArray);
DisplayArray(myArray);
String[] myOtherArray =
{
"We", "Hold", "These", "Truths",
"To", "Be", "Self", "Evident",
};
Console.WriteLine("Display myOtherArray...");
DisplayArray(myOtherArray);
Console.WriteLine("Sort and display myOtherArray...");
Array.Sort(myOtherArray);
DisplayArray(myOtherArray);
}
[STAThread]
static void Main()
{
TesterReverseAndSort t = new TesterReverseAndSort();
t.Run();
}
}
}
Related examples in the same category