illustrates member accessibility
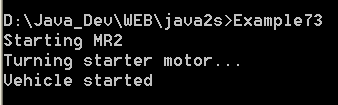
/*
Mastering Visual C# .NET
by Jason Price, Mike Gunderloy
Publisher: Sybex;
ISBN: 0782129110
*/
/*
Example7_3.cs illustrates member accessibility
*/
using System;
// declare the MotorVehicle class
class MotorVehicle
{
// declare the fields
private string make;
protected string model;
// define a constructor
public MotorVehicle(string make, string model)
{
this.make = make;
this.model = model;
}
// define the Start() method (may be overridden in a
// derived class)
public virtual void Start()
{
TurnStarterMotor();
System.Console.WriteLine("Vehicle started");
}
// define the TurnStarterMotor() method
private void TurnStarterMotor()
{
System.Console.WriteLine("Turning starter motor...");
}
}
// declare the Car class (derived from MotorVehicle)
class Car : MotorVehicle
{
// define a constructor
public Car(string make, string model) :
base(make, model)
{
// do nothing
}
// override the base class Start() method
public override void Start()
{
Console.WriteLine("Starting " + model); // model accessible
base.Start(); // calls the Start() method in the base class
// Console.WriteLine("make = " + make); // make is not accessible
}
}
public class Example7_3
{
public static void Main()
{
// create a Car object and call the object's Accelerate() method
Car myCar = new Car("Toyota", "MR2");
myCar.Start();
// make and model are not accessible, so the following two lines
// are commented out
// Console.WriteLine("myCar.make = " + myCar.make);
// Console.WriteLine("myCar.model = " + myCar.model);
}
}
Related examples in the same category