Interfaces:Working with Interfaces
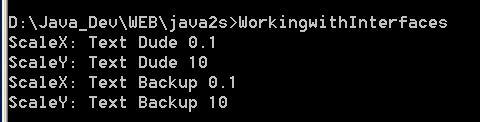
/*
A Programmer's Introduction to C# (Second Edition)
by Eric Gunnerson
Publisher: Apress L.P.
ISBN: 1-893115-62-3
*/
// 10 - Interfaces\Working with Interfaces
// copyright 2000 Eric Gunnerson
using System;
public class WorkingwithInterfaces
{
public static void Main()
{
DiagramObject[] dArray = new DiagramObject[100];
dArray[0] = new DiagramObject();
dArray[1] = new TextObject("Text Dude");
dArray[2] = new TextObject("Text Backup");
// array gets initialized here, with classes that
// derive from DiagramObject. Some of them implement
// IScalable.
foreach (DiagramObject d in dArray)
{
if (d is IScalable)
{
IScalable scalable = (IScalable) d;
scalable.ScaleX(0.1F);
scalable.ScaleY(10.0F);
}
}
}
}
interface IScalable
{
void ScaleX(float factor);
void ScaleY(float factor);
}
public class DiagramObject
{
public DiagramObject() {}
}
public class TextObject: DiagramObject, IScalable
{
public TextObject(string text)
{
this.text = text;
}
// implementing IScalable.ScaleX()
public void ScaleX(float factor)
{
Console.WriteLine("ScaleX: {0} {1}", text, factor);
// scale the object here.
}
// implementing IScalable.ScaleY()
public void ScaleY(float factor)
{
Console.WriteLine("ScaleY: {0} {1}", text, factor);
// scale the object here.
}
private string text;
}
Related examples in the same category