Illustrates abstract classes and methods
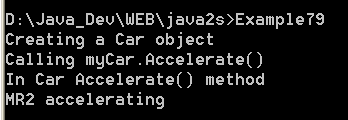
/*
Mastering Visual C# .NET
by Jason Price, Mike Gunderloy
Publisher: Sybex;
ISBN: 0782129110
*/
/*
Example7_9.cs illustrates abstract classes and methods
*/
using System;
// declare the abstract MotorVehicle class
abstract class MotorVehicle
{
// declare the fields
public string make;
public string model;
// define a constructor
public MotorVehicle(string make, string model)
{
this.make = make;
this.model = model;
}
// declare the abstract Accelerate() method (no code)
abstract public void Accelerate();
}
// declare the Car class (derived from MotorVehicle)
class Car : MotorVehicle
{
// define a constructor
public Car(string make, string model) :
base(make, model)
{
// do nothing
}
// override the Accelerate() method (contains code)
public override void Accelerate()
{
Console.WriteLine("In Car Accelerate() method");
Console.WriteLine(model + " accelerating");
}
}
public class Example7_9
{
public static void Main()
{
// create a Car object
Console.WriteLine("Creating a Car object");
Car myCar = new Car("Toyota", "MR2");
// call the Car object's Accelerate() method
Console.WriteLine("Calling myCar.Accelerate()");
myCar.Accelerate();
}
}
Related examples in the same category