Demonstrates the use of a virtual property to override a base class property
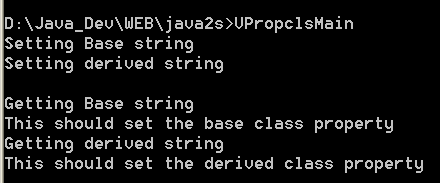
/*
C# Programming Tips & Techniques
by Charles Wright, Kris Jamsa
Publisher: Osborne/McGraw-Hill (December 28, 2001)
ISBN: 0072193794
*/
//
// VProp.cs -- Demonstrates the use of a virtual method to override
// a base class method.
//
// Compile this program with the following command line:
// C:>csc VProp.cs
namespace nsVirtual
{
using System;
public class VPropclsMain
{
static public void Main ()
{
clsBase Base = new clsBase();
clsFirst First = new clsFirst();
Base.SetString ("This should set the base class property");
First.SetString ("This should set the derived class property");
Console.WriteLine();
Console.WriteLine (Base.GetString());
Console.WriteLine (First.GetString());
}
}
class clsBase
{
public void SetString (string str)
{
StrProp = str;
}
public string GetString ()
{
return (StrProp);
}
virtual protected string StrProp
{
get
{
Console.WriteLine ("Getting Base string");
return (m_BaseString);
}
set
{
Console.WriteLine ("Setting Base string");
m_BaseString = value;
}
}
private string m_BaseString = "";
}
class clsFirst : clsBase
{
override protected string StrProp
{
get
{
Console.WriteLine ("Getting derived string");
return (m_DerivedString);
}
set
{
Console.WriteLine ("Setting derived string");
m_DerivedString = value;
}
}
private string m_DerivedString = "";
}
}
Related examples in the same category