Translate Transform
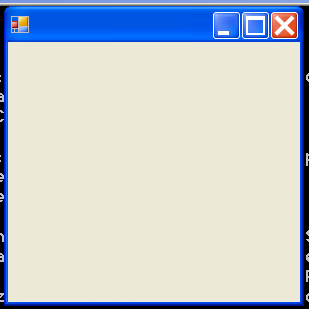
/*
User Interfaces in C#: Windows Forms and Custom Controls
by Matthew MacDonald
Publisher: Apress
ISBN: 1590590457
*/
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Drawing.Drawing2D;
namespace GDI_Basics
{
/// <summary>
/// Summary description for TranslateTransform.
/// </summary>
public class TranslateTransform : System.Windows.Forms.Form
{
/// <summary>
/// Required designer variable.
/// </summary>
private System.ComponentModel.Container components = null;
public TranslateTransform()
{
//
// Required for Windows Form Designer support
//
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent call
//
}
/// <summary>
/// Clean up any resources being used.
/// </summary>
protected override void Dispose( bool disposing )
{
if( disposing )
{
if(components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
#region Windows Form Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
//
// TranslateTransform
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 13);
this.ClientSize = new System.Drawing.Size(292, 266);
this.Name = "TranslateTransform";
this.Text = "TranslateTransform";
this.Paint += new System.Windows.Forms.PaintEventHandler(this.TranslateTransform_Paint);
}
#endregion
private void TranslateTransform_Paint(object sender, System.Windows.Forms.PaintEventArgs e)
{
// Draw several squares in different places.
DrawRectangle(e.Graphics);
e.Graphics.TranslateTransform(180, 60);
DrawRectangle(e.Graphics);
e.Graphics.TranslateTransform(-50, 80);
DrawRectangle(e.Graphics);
e.Graphics.TranslateTransform(-100, 50);
DrawRectangle(e.Graphics);
}
private void DrawRectangle(Graphics g)
{
Pen drawingPen = new Pen(Color.Red, 30);
// Draw a rectangle at a fixed position.
g.DrawRectangle(drawingPen, new Rectangle(20, 20, 20, 20));
}
[STAThread]
static void Main()
{
Application.Run(new TranslateTransform());
}
}
}
Related examples in the same category