Gradient Label
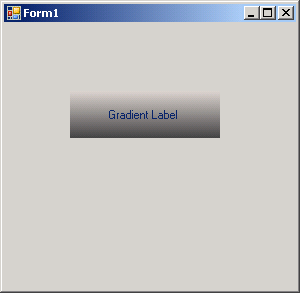
/*
Code revised from chapter 3
GDI+ Custom Controls with Visual C# 2005
By Iulian Serban, Dragos Brezoi, Tiberiu Radu, Adam Ward
Language English
Paperback 272 pages [191mm x 235mm]
Release date July 2006
ISBN 1904811604
Sample chapter
http://www.packtpub.com/files/1604_CustomControls_SampleChapter.pdf
For More info on GDI+ Custom Control with Microsoft Visual C# book
visit website www.packtpub.com
*/
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Text;
using System.Windows.Forms;
using System.Drawing.Drawing2D;
namespace GradientLabelTest00
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private System.ComponentModel.IContainer components = null;
protected override void Dispose(bool disposing)
{
if (disposing && (components != null))
{
components.Dispose();
}
base.Dispose(disposing);
}
#region Windows Form Designer generated code
private void InitializeComponent()
{
this.gradientLabel1 = new GradientLabelTest00.GradientLabel();
this.SuspendLayout();
this.gradientLabel1.BackColor = System.Drawing.SystemColors.ControlLight;
this.gradientLabel1.BackColor2 = System.Drawing.SystemColors.ControlDarkDark;
this.gradientLabel1.ForeColor = System.Drawing.SystemColors.ActiveCaption;
this.gradientLabel1.Location = new System.Drawing.Point(66, 68);
this.gradientLabel1.Name = "gradientLabel1";
this.gradientLabel1.Size = new System.Drawing.Size(150, 47);
this.gradientLabel1.TabIndex = 0;
this.gradientLabel1.Text = "Gradient Label";
//
// Form1
//
this.AutoScaleDimensions = new System.Drawing.SizeF(6F, 13F);
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.ClientSize = new System.Drawing.Size(292, 266);
this.Controls.Add(this.gradientLabel1);
this.Name = "Form1";
this.Text = "Form1";
this.ResumeLayout(false);
}
#endregion
private GradientLabel gradientLabel1;
/// <summary>
/// The main entry point for the application.
/// </summary>
[STAThread]
static void Main()
{
Application.EnableVisualStyles();
Application.SetCompatibleTextRenderingDefault(false);
Application.Run(new Form1());
}
}
public partial class GradientLabel : Control
{
private Color backColor2 = SystemColors.ControlLight;
public GradientLabel()
{
InitializeComponent();
}
public Color BackColor2
{
get
{
return backColor2;
}
set
{
if (backColor2 != value)
{
backColor2 = value;
Invalidate();
}
}
}
private void GradientLabel_Paint(object sender, PaintEventArgs e)
{
}
protected override void OnPaint(PaintEventArgs e)
{
base.OnPaint(e);
LinearGradientBrush brush = new LinearGradientBrush(new Point(0, 0), new Point(0, Height), BackColor, BackColor2);
e.Graphics.FillRectangle(brush, ClientRectangle);
Brush foreBrush = new SolidBrush(ForeColor);
SizeF textSize = e.Graphics.MeasureString(Text, Font);
e.Graphics.DrawString(Text, Font, foreBrush, (Width - textSize.Width) / 2, (Height - textSize.Height) / 2);
brush.Dispose();
foreBrush.Dispose();
}
private System.ComponentModel.IContainer components = null;
protected override void Dispose(bool disposing)
{
if (disposing && (components != null))
{
components.Dispose();
}
base.Dispose(disposing);
}
#region Component Designer generated code
private void InitializeComponent()
{
this.SuspendLayout();
this.BackColor = System.Drawing.SystemColors.ControlDarkDark;
this.ForeColor = System.Drawing.SystemColors.HotTrack;
this.Size = new System.Drawing.Size(150, 24);
this.Text = "Gradient Label";
this.Paint += new System.Windows.Forms.PaintEventHandler(this.GradientLabel_Paint);
this.ResumeLayout(false);
}
#endregion
}
}
Related examples in the same category