Drag and drop shape
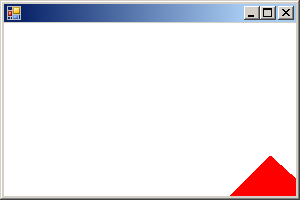
using System.Drawing;
using System.Drawing.Drawing2D;
using System.Windows.Forms;
public class TryMouse : Form {
private Region region;
private int oldX = 0;
private int oldY = 0;
public TryMouse() {
Size = new Size(300,200);
BackColor = Color.White;
GraphicsPath p = new GraphicsPath();
p.AddPolygon(new Point[]{new Point(50,10), new Point(10,50), new Point(150,100)});
region = new Region(p);
}
protected override void OnPaint(PaintEventArgs e) {
Graphics g = e.Graphics;
g.FillRegion(Brushes.Red, region);
base.OnPaint(e);
}
protected override void OnMouseDown(MouseEventArgs e) {
if (region.GetBounds(CreateGraphics()).Contains(e.X, e.Y)){
oldX = e.X;
oldY = e.Y;
}
base.OnMouseDown(e);
}
protected override void OnMouseUp(MouseEventArgs e) {
region.Translate(e.X-oldX, e.Y-oldY);
oldX = e.X;
oldY = e.Y;
Invalidate();
base.OnMouseUp(e);
}
public static void Main() {
Application.Run(new TryMouse());
}
}
Related examples in the same category