Clipping
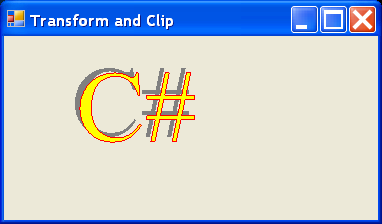
/*
Professional Windows GUI Programming Using C#
by Jay Glynn, Csaba Torok, Richard Conway, Wahid Choudhury,
Zach Greenvoss, Shripad Kulkarni, Neil Whitlow
Publisher: Peer Information
ISBN: 1861007663
*/
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
using System.Drawing.Drawing2D;
namespace Clipping
{
/// <summary>
/// Summary description for Clipping.
/// </summary>
public class Clipping : System.Windows.Forms.Form
{
/// <summary>
/// Required designer variable.
/// </summary>
private System.ComponentModel.Container components = null;
GraphicsPath gP;
public Clipping()
{
//
// Required for Windows Form Designer support
//
InitializeComponent();
this.Text = "Transform and Clip";
//
// TODO: Add any constructor code after InitializeComponent call
//
}
/// <summary>
/// Clean up any resources being used.
/// </summary>
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
#region Windows Form Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
//
// Clipping
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 13);
this.ClientSize = new System.Drawing.Size(292, 149);
this.Name = "Clipping";
this.Text = "Clipping";
}
#endregion
/// <summary>
/// The main entry point for the application.
/// </summary>
[STAThread]
static void Main()
{
Application.Run(new Clipping());
}
protected override void OnPaint(System.Windows.Forms.PaintEventArgs e)
{
Graphics g = e.Graphics;
DrawPathTransform(g);
//DrawClip(g);
g.Dispose();
}
void AddStringToPath(string s)
{
int fSt = (int)FontStyle.Regular;
Point xy = new Point(50, 10);
FontFamily fF = new FontFamily("Times new roman");
StringFormat sFr = StringFormat.GenericDefault;
gP.AddString(s, fF, fSt, 100, xy, sFr); // add the string to the path
}
void DrawPathTransform(Graphics g)
{
gP = new GraphicsPath(); // create a new path
AddStringToPath("C#");
g.FillPath(Brushes.Gray, gP); // draw the path to the surface
Matrix m = new Matrix();
m.Translate(5, 5);
gP.Transform(m);
g.FillPath(Brushes.Yellow, gP); // draw the path to the surface
g.DrawPath(Pens.Red, gP); // draw the path to the surface
}
void DrawClip(Graphics g)
{
gP = new GraphicsPath(); // create a new path
AddStringToPath("C#");
g.SetClip(gP);
g.TranslateClip(-5, -5);
RectangleF rc = gP.GetBounds();
rc.Offset(-5, -5);
g.FillRectangle(Brushes.Gray, rc); // results in gray C#
g.ResetClip();
g.FillPath(Brushes.Yellow, gP); // draw the path to the surface
g.DrawPath(Pens.Red, gP); // draw the path to the surface
}
}
}
Related examples in the same category