All Line Join
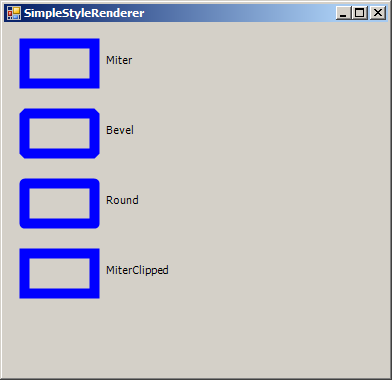
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Text;
using System.Windows.Forms;
using System.Windows.Forms.VisualStyles;
using System.Drawing.Drawing2D;
public class Form1 : Form
{
public Form1() {
InitializeComponent();
}
private void SimpleStyleRenderer_Paint(object sender, PaintEventArgs e)
{
e.Graphics.SmoothingMode = SmoothingMode.AntiAlias;
Pen myPen = new Pen(Color.Blue, 10);
int y = 20;
foreach (LineJoin join in Enum.GetValues(typeof(LineJoin)))
{
myPen.LineJoin = join;
e.Graphics.DrawRectangle(myPen, 20, y, 70, 40);
e.Graphics.DrawString(join.ToString(), new Font("Tahoma", 8), Brushes.Black, 100, y + 10);
y += 70;
}
}
private void InitializeComponent()
{
this.SuspendLayout();
//
// SimpleStyleRenderer
//
this.AutoScaleDimensions = new System.Drawing.SizeF(6F, 13F);
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.ClientSize = new System.Drawing.Size(384, 353);
this.Name = "SimpleStyleRenderer";
this.Text = "SimpleStyleRenderer";
this.Paint += new System.Windows.Forms.PaintEventHandler(this.SimpleStyleRenderer_Paint);
this.ResumeLayout(false);
}
[STAThread]
static void Main()
{
Application.EnableVisualStyles();
Application.Run(new Form1());
}
}
Related examples in the same category