Daisy chaining the horses both ways
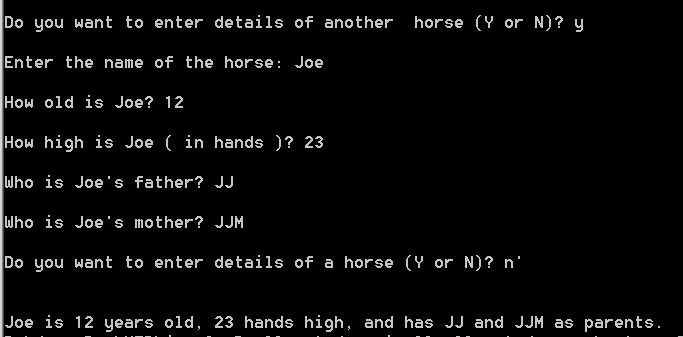
#include <stdio.h>
#include <ctype.h>
#include <stdlib.h>
struct cat
{
int age;
int height;
char name[20];
char father[20];
char mother[20];
struct cat *next; /* Pointer to next structure */
struct cat *previous; /* Pointer to previous structure */
};
int main()
{
struct cat *first = NULL;
struct cat *current = NULL;
struct cat *last = NULL;
char test = '\0';
for( ; ; ) {
printf("\nDo you want to enter details of a%s cat (Y or N)? ", first == NULL?"nother " : "");
scanf(" %c", &test );
if(tolower(test) == 'n')
break;
/* Allocate memory for each new cat structure */
current = (struct cat*)malloc(sizeof(struct cat));
if( first == NULL )
{
first = current; /* Set pointer to first cat */
current->previous = NULL;
}
else
{
last->next = current; /* Set next address for previous cat */
current->previous = last; /* Previous address for current cat */
}
printf("\nEnter the name of the cat: ");
scanf("%s", current -> name ); /* Read the cat's name */
printf("\nHow old is %s? ", current -> name);
scanf("%d", ¤t -> age); /* Read the cat's age */
printf("\nHow high is %s ( in hands )? ", current -> name);
scanf("%d", ¤t -> height); /* Read the cat's height */
printf("\nWho is %s's father? ", current -> name);
scanf("%s", current -> father); /* Get the father's name */
printf("\nWho is %s's mother? ", current -> name);
scanf("%s", current -> mother); /* Get the mother's name */
current -> next = NULL; /* In case its the last cat...*/
last = current; /* Save address of last cat */
}
while(current != NULL) /* Output cat data in reverse order */
{
printf("\n\n%s is %d years old, %d hands high,",
current->name, current->age, current->height);
printf(" and has %s and %s as parents.", current->father,
current->mother);
last = current; /* Save pointer to enable memory to be freed */
current = current->previous; /* current points to previous in list */
free(last); /* Free memory for the cat we output */
}
}
Related examples in the same category