Defining a macro to compare two values
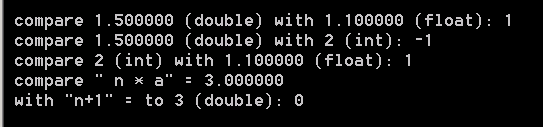
/*
The macro function can work with numerical values
of any type. The values can be variables, constants or expressions.
*/
#include <stdio.h>
#define COMPARE(x,y) ((x)<(y)?-1:((x)==(y)?0:1))
int main()
{
double a = 1.5;
int n = 2;
float z = 1.1f;
printf("\n compare %lf (double) with %f (float): %d", a , z, COMPARE(a,z));
printf("\n compare %lf (double) with %d (int): %d", a , n, COMPARE(a,n));
printf("\n compare %d (int) with %f (float): %d", n , z, COMPARE(n,z));
printf("\n compare \" n * a\" = %lf \n"
" with \"n+1\" = to %d (double): %d",
n*a , n+1, COMPARE(n*a,n+1));
printf("\n");
}
Related examples in the same category